This blog provides a simple example of using PowerShell select-string to match regular expressions.
Select-string and regular expressions come in really useful when we want to extract specific data from a string that matches a particular pattern. Consider this URL:
https://www.alkanesolutions.co.uk/2023/05/05/another-great-blog/
We can see that it contains the date in year/month/day. But how can we extract it easily? We can use regular expressions. And the expression we’ll use in this example is:
\d{4}/\d{2}/\d{2}
Splitting this up, we are simply searching for a pattern that matches 4 {4}
digits \d
followed by a forward slash, then 2 {2}
digits \d
followed by a forward slash, then 2 {2}
digits \d
.
We need to expand on this though, because when we search our string we want to be able to extract the day, the month or the year separately. And to do this we need to make each part a matching group. We can do this by simply enclosing each matching group in a rounded bracket like so:
(\d{4})/(\d{2})/(\d{2})
So if we find matches in our string, the first matching group will be the whole pattern (\d{4})/(\d{2})/(\d{2})
, the second matching group will be (\d{4})
, the third (\d{2})
and finally the fourth (\d{2})
.
Here’s a quick example:
$text = "https://www.alkanesolutions.co.uk/2023/05/05/another-great-blog/"
$text | Select-String -Pattern "(\d{4})/(\d{2})/(\d{2})" | Select -Expand Matches | Select -Expand Groups
and the result is:
Groups : {0, 1, 2, 3}
Success : True
Name : 0
Captures : {0}
Index : 34
Length : 10
Value : 2023/05/05
Success : True
Name : 1
Captures : {1}
Index : 34
Length : 4
Value : 2023
Success : True
Name : 2
Captures : {2}
Index : 39
Length : 2
Value : 05
Success : True
Name : 3
Captures : {3}
Index : 42
Length : 2
Value : 05
We can clearly see that there are 4 groups captured in total as mentioned above, and you can see the value of each matching group. This is handy because it makes it really easy to extract the data we want like so:
$text = "https://www.alkanesolutions.co.uk/2023/05/05/another-great-blog/"
$matchingGroups = $text | Select-String -Pattern "(\d{4})/(\d{2})/(\d{2})" | Select -Expand Matches | Select -Expand Groups
$wholeDate = $matchingGroups | Where Name -eq 0 | Select -Expand Value
$justYear = $matchingGroups | Where Name -eq 1 | Select -Expand Value
$justMonth = $matchingGroups | Where Name -eq 2 | Select -Expand Value
$justDay = $matchingGroups | Where Name -eq 3 | Select -Expand Value
write-host $wholeDate
write-host $justYear
write-host $justMonth
write-host $justDay
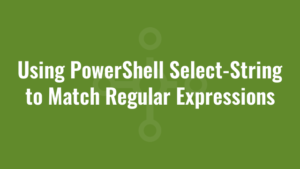
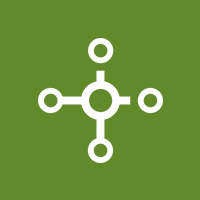