Using SharePoint with the SPServices library provides an excellent way of using jQuery to read from a SharePoint lists using client-side code.
Using SharePoint with SPServices
SPServices is a great library for use with SharePoint.
I’ll show you a quick example of how we can use it to read from a SharePoint list as part of validating new or existing list items.
Prerequisite Knowledge
You will need to have a basic understanding of HTML, and browsing the DOM structure using the browser’s developer tools. You will also need to have a basic understanding of CAML query syntax and jQuery.
Example: Check for Duplicate Items with SPServices
When we want to add a new item to our SharePoint list, we want to ensure that it isn’t a duplicate. Coupled with this, we also want to make sure that when users edit a list item they don’t create a duplicate item too! So to do this we need to add some validation.
First you need to download and install a copy of SharePoint Designer.
Once installed, run it up and connect to your SharePoint site (you will need the appropriate permissions to do this). You will simply click ‘Open Site’ and enter the URL. As an example, if the URL for your list is:
https://alkane.sharepoint.com/alkanehome/Lists/alkane%20tracker/AllItems.aspx
You will open the site:
https://alkane.sharepoint.com/alkanehome/
Once opened, click on Lists and Libraries. Then click on your list. Under the Forms section click on the form you wish to edit.
- NewForm.aspx is the form you see when you create a new entry.
- EditForm.aspx is the form you see when you edit a record.
- DispForm.aspx is usually the read-only form view of the record. We shouldn’t need to edit this for now.
In our example, we’d probably want to make changes to both the NewForm.aspx and the EditForm.aspx.
Select NewForm.aspx and put the SharePoint form into Advanced Mode.
Find the tag called
<asp:Content ContentPlaceHolderId="PlaceHolderAdditionalPageHead" runat="server">
and just before its closing
</asp:Content>
tag we can start adding the following code:
Firstly add a link to the external jQuery and SPServices libraries hosted on a CDN:
<!--jQuery library-->
<script type="text/javascript" src="https://code.jquery.com/jquery-2.2.4.min.js"></script>
<!--SPServices library-->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery.SPServices/2014.02/jquery.SPServices-2014.02.min.js"></script>
And below this we add the validation code – I have commented inline so you can understand the logic:
<script type="text/javascript">
//function to get a SharePoint form element by its Title attribute
//there's probably a more elegant way to do this
function getElementByTitle(elementTitle, elementType)
{
return $(elementType + "[title='" + elementTitle + "']");
}
//function to get a SharePoint form element by the start of the ID attribute
//there's probably a more elegant way to do this
function getElementByIDStart(elementID)
{
return $("[id^='" + elementID + "']");
}
function checkIfItemExists()
{
//get the value of the entries in our form that we want to check
var appVendor = getElementByTitle('Application Vendor Required Field','input').val();
var appName = getElementByTitle('Application Name Required Field','input').val();
//get the ID of the current form item
var thisItemId = GetUrlKeyValue('ID');
//construct a CAML query to return all items that match this vendor name, application name but NOT the same ID (in case we are in edit mode)
var existingItemQuery =
"<Query>" +
"<Where>" +
"<And>" +
"<And>" +
"<Eq>" +
"<FieldRef Name='Application_x0020_Vendor' /><Value Type='Text' >" + appVendor + "</Value>" +
"</Eq>" +
"<Eq>" +
"<FieldRef Name='Application_x0020_Name' /><Value Type='Text' >" + appName + "</Value>" +
"</Eq>" +
"</And>" +
"<Neq>" +
"<FieldRef Name='ID' /><Value Type='Text' >" + thisItemId + "</Value>" +
"</Neq>" +
"</And>" +
"</Where>" +
"</Query>";
//initialise our item found count to zero
var ItemCount = 0;
//user SPServices to 'GetListItems' of the 'Alkane Tracker' list, passing our CAML query
$().SPServices({
operation: "GetListItems",
async: false,
listName: "Alkane Tracker",
CAMLQuery: existingItemQuery,
CAMLViewFields: "<ViewFields><FieldRef Name='Application_x0020_Name' /></ViewFields>",
completefunc: function (xData, Status) {
$(xData.responseXML).SPFilterNode("z:row").each(function() {
ItemCount = ItemCount + 1;
});
}
});
//if we have found 1 or more items then it already exists and is a duplicate
if (ItemCount > 0)
{
alert("The proposed item already exists!");
return false;
}
return true;
}
//SharePoint checks if PreSaveItems return false. In this case it will not allow the form to submit.
var PreSaveItem = function() {
var itemDoesNotExist = checkIfItemExists();
return (itemDoesNotExist);
}
</script>
That should be pretty much it for our basic example, so save your form and try it out. You’ll also want to paste the same code into EditForm.aspx too.
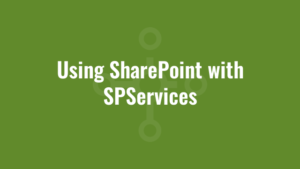
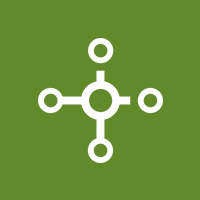