This post explains how to export to CSV using PowerShell and dynamic data. I find that hashtables are quite useful for various scenarios when scripting in PowerShell. We can also utilise them when exporting data using Export-CSV.
I provide two approaches to this. Both approaches work by adding hashtables to an array. By default if you export an array of hashtables to an array you will lose the column ordering. With PowerShell 2 we can circumvent this issue by using ‘Select’ and specifying the order of the columns we would like to select:
$csvnamePS2 = "C:\Temp\" + (Get-Date -format "dd-MM-yyyy-HH-mm") + ".csv"
$global:csvarrayPS2 = @()
function Export-To-CSV()
{
param (
[string]$FirstName,
[string]$LastName
)
$wrapper = New-Object -TypeName PSObject -Property @{ FirstName = $FirstName; LastName = $LastName;} | Select FirstName, LastName
$global:csvarrayPS2 += $wrapper
}
Export-To-CSV "Captain" "Hook"
Export-To-CSV "Peter" "Pan"
$global:csvarrayPS2 | Export-Csv -NoTypeInformation -Path $csvnamePS2
With PowerShell 3 we can simplify this, by specifying the [ordered] type for our hashtable:
$csvnamePS3 = "C:\Temp\" + (Get-Date -format "dd-MM-yyyy-HH-mm") + ".csv"
$global:csvarrayPS3 = @()
function Export-To-CSV()
{
param (
[string]$FirstName,
[string]$LastName
)
$wrapper = [ordered]@{ FirstName = $FirstName; LastName = $LastName;}
$global:csvarrayPS3 += New-Object psobject -property $wrapper
}
Export-To-CSV "Captain" "Hook"
Export-To-CSV "Peter" "Pan"
$global:csvarrayPS3 | Export-Csv -NoTypeInformation -Path $csvnamePS3
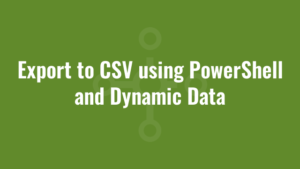
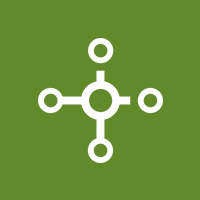