There are a couple of ways of using PowerShell to replace strings. We can use either the Replace() method or the -replace operator.
PowerShell String Replacement using the Replace() Method
This isn’t my favourite approach to replacing string using PowerShell, since it is case sensitive. What this means is that if you want to replace the word “red” with “blue”, it will NOT replace the word “Red” due to it being a different case (Upper case ‘R’ in case you didn’t notice!)
#string to search through
$stringToSearch = "the red fox and the Red cat"
#replace the word 'red' for 'blue'
$stringAfterReplace = $stringToSearch.Replace("red","blue")
#output result
write-host $stringAfterReplace
#result
#the blue fox and the Red cat
Instead, I prefer to use the replace operator since it is case-insensitive and a lot more powerful.
PowerShell String Replacement using the -Replace Operator
In this example, when we talk about performing a case-insensitive replace it means we want to replace instances of “red”, “Red”, “reD” or otherwise with the word “blue”. Notice the slightly different syntax:
#string to search through
$stringToSearch = "the red fox and the Red cat"
#replace the word 'red' for 'blue'
$stringAfterReplace = $stringToSearch -replace "red","blue"
#output result
write-host $stringAfterReplace
#result
#the blue fox and the blue cat
The -replace operator also has a ‘sister’ operator called -creplace that gives us the advantage of behaving like the Replace() method – the prefixed ‘c’ meaning perform a ‘c’ase sensitive replace:
#string to search through
$stringToSearch = "the red fox and the Red cat"
#replace the word 'red' for 'blue'
$stringAfterReplace = $stringToSearch -creplace "red","blue"
#output result
write-host $stringAfterReplace
#result
#the blue fox and the Red cat
The final (and biggest) advantage of using the -replace operator for PowerShell string replacement is that it uses rather complicated yet powerful regular expressions! Without getting too detailed (because regular expressions are a huge topic) take this example where we want to replace all occurrences of digits that are 2 in length (i.e 75 and NOT 9) with the number 10:
$stringToSearch = "the red fox is 75 and the Red cat is 9"
$stringAfterReplace = $stringToSearch -replace "\d{2}","10"
write-host $stringAfterReplace
#result
#the red fox is 10 and the Red cat is 9
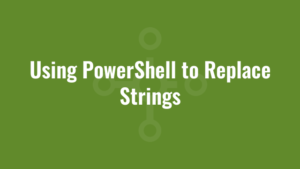
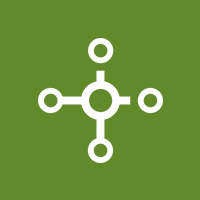