Here we explain how to use PowerShell to read from a text file, and iterate through it line by line.
Consider the following text file called c:\alkane.txt:
line 1
line 2
line 3
line 4
line 5
We can simply read from this and output the content like so:
$content = get-content C:\alkane.txt
write-host $content
This actually returns an array of strings into the $content variable. And writing this out to the terminal looks like this: line 1 line 2 line 3 line 4 line 5
Since it’s an array of strings, we could access each line individually like so:
write-host $content[0]
#prints line 1
write-host $content[1]
#prints line 2
write-host $content[2]
#prints line 3
Or if we wanted to read the content line by line, we could use a For loop like so:
$content = get-content C:\alkane.txt
#method 1
foreach($line in $content) {
write-host $line
}
#method 2, so we know line number
for($i=0; $i -lt $content.Count; $i++) {
write-host $content[$i]
}
We may only want to return the first, say 3, lines of data like so:
$content = get-content C:\alkane.txt -totalcount 3
foreach($line in $content) {
write-host $line
}
Which returns:
line 1
line 2
line 3
Or the last 3 lines of data like so:
$content = get-content C:\alkane.txt -tail 3
foreach($line in $content) {
write-host $line
}
Which returns:
line 3
line 4
line 5
Alternatively if we just wanted to print the contents to the screen whilst retaining newline characters, we can use the -raw parameter like so:
$content = get-content C:\alkane.txt -raw
write-host $content
which produces the following output:
line 1
line 2
line 3
line 4
line 5
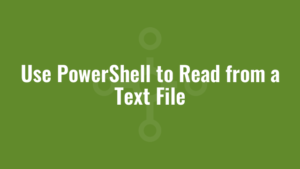
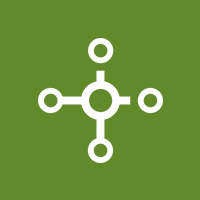