This blog post explains how we can launch a process using PowerShell and Start-Process.
In its most simplistic form, we can launch a process and essentially forget about it. In this example we’re going to launch Google Chrome. You will note by reading the output that it will launch Chrome and carry on with the rest of our script without waiting.
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
write-host "Launching $pathToChrome"
Start-Process -FilePath $pathToChrome
write-host "Finished"
Maybe we want some user input to complete a form? So we need to specify an argument to the executable we are launching. In this example, we are passing a web address to Chrome so the browser opens on our website:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
Start-Process -FilePath $pathToChrome -ArgumentList $arguments
write-host "Finished"
You’ll notice that script execution continues after we launch Chrome. But what if we want to wait for the user to complete a web-based form and close the browser? We can simply add the -Wait parameter to Start-Process like so:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
Start-Process -FilePath $pathToChrome -ArgumentList $arguments -Wait
write-host "Finished"
And since the -ArgumentList parameter is an array of strings, we can pass multiple arguments to our executable. In this example we are opening the Alkane Solutions website in full screen mode:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "--start-fullscreen","https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
Start-Process -FilePath $pathToChrome -ArgumentList $arguments -Wait
write-host "Finished"
In many instances we want to launch and executable and check for its exit code – usually an exit code of 0 represents a success, and any other exit code represents a failure of some sort.
To retrieve the exit code of our launched process, we must use the
-Wait
and the
-PassThru
parameter like so:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "--start-fullscreen","https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
$exitCodeObject = Start-Process -FilePath $pathToChrome -ArgumentList $arguments -Wait -PassThru
$exitCode = $exitCodeObject.ExitCode
write-host "Exit code is $exitCode"
write-host "Finished"
We also posted an example previously of how we can install and uninstall windows installer MSI files using Start-Process in a similar way.
We can also request that the executable is “Run As Administrator” by specifying the RunAs verb.
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "--start-fullscreen","https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
$exitCodeObject = Start-Process -FilePath $pathToChrome -ArgumentList $arguments -Wait -PassThru -Verb RunAs
$exitCode = $exitCodeObject.ExitCode
write-host "Exit code is $exitCode"
write-host "Finished"
If you launch it from a “normal” user account you will be prompted to enter credentials. To find out which verbs you can use when running a process, you can utilise the following command:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$startExe = New-Object System.Diagnostics.ProcessStartInfo -Args $pathToChrome
$startExe.verbs
Finally, we can request that a process is launched as another user. To do this, we need to prompt the user for credentials by using
Get-Credential
. And we can pass the output of this to
Start-Process
using the
-Credential
parameter like so:
$pathToChrome = "$env:ProgramFiles\Google\Chrome\Application\Chrome.exe"
$arguments = "--start-fullscreen","https://www.alkanesolutions.co.uk"
write-host "Launching $pathToChrome"
write-host "Requesting credentials"
$cred = Get-Credential
if ($cred -ne $null) {
$exitCodeObject = Start-Process -FilePath $pathToChrome -ArgumentList $arguments -Wait -PassThru -Credential $cred
$exitCode = $exitCodeObject.ExitCode
write-host "Exit code is $exitCode"
}
write-host "Finished"
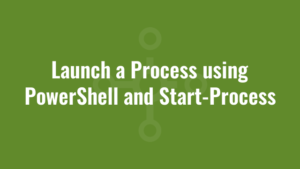
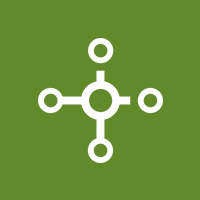