We can use PowerShell to calculate bit flags, so that we know which configurations have been set. For examples where we don’t want to create our own enum, we can read using PowerShell bitwise comparison operators to check and set bit flags.
Take the following example – I am reading a decimal value called OfferFlags on the SMS_ApplicationAssignment WMI Class and the value of the field can be a combination of the following bit flags:
Value | Offer flag |
---|---|
1 | PREDEPLOY |
2 | ONDEMAND |
4 | ENABLEPROCESSTERMINATION |
8 | ALLOWUSERSTOREPAIRAPP |
16 | RELATIVESCHEDULE |
32 | HIGHIMPACTDEPLOYMENT |
For example, if the value of the OfferFlag was 44 we can tell from the table above that this setting includes ‘HIGHIMPACTDEPLOYMENT’ (32) with ‘ALLOWUSERSTOREPAIRAPP’ (8) and ‘ENABLEPROCESSTERMINATION’ (4) since 32 + 8 + 4 = 44!
Use PowerShell to Calculate Bit Flags
But how can we check which bits are set programmatically? We can use a handy enum statement introduced in PowerShell 5.1 to create a strongly typed set of labels that represents the documented bit flags above:
#create an enum and replicate bit flags in documentation
[flags()]
enum SMS_ApplicationAssignment_OfferFlags
{
None = 0
PREDEPLOY = 1
ONDEMAND = 2
ENABLEPROCESSTERMINATION = 4
ALLOWUSERSTOREPAIRAPP = 8
RELATIVESCHEDULE = 16
HIGHIMPACTDEPLOYMENT = 32
}
#set the value to an example for this demo - 44 in this case
[SMS_ApplicationAssignment_OfferFlags]$assignmentFlags = 44
#simply print out all flags that are set like so
write-host $assignmentFlags
#or check for individual flag sets like so
if ($assignmentFlags.HasFlag([SMS_ApplicationAssignment_OfferFlags]::HIGHIMPACTDEPLOYMENT)) {
write-host "HIGHIMPACTDEPLOYMENT is set"
}
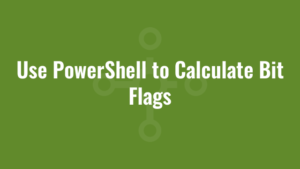
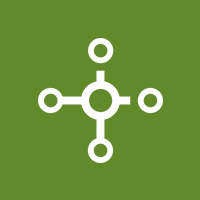