There are multiple methods to check if a file exists using PowerShell. In this blog post, we’ll explore two common approaches: using the
Test-Path
cmdlet and utilising .NET methods.
Using the Test-Path Cmdlet to Check if a File Exists
The
Test-Path
cmdlet is a straightforward and effective way to check if a file exists in Windows PowerShell. It returns
$true
if the file exists and
$false
if it doesn’t. Here’s a basic example:
$filePath = "C:\Alkane\Alkane.txt"
if (Test-Path $filePath) {
Write-Host "File exists at $filePath"
} else {
Write-Host "File does not exist at $filePath"
}
In this example, we assign the file path to the
$filePath
variable and use
Test-Path
to check its existence. The script then prints an appropriate message based on the result.
Using .NET Methods to Check if a File Exists
Another way to check if a file exists in Windows PowerShell is by utilising .NET methods. You can use the
[System.IO.File]::Exists()
method to achieve this. Here’s an example:
$filePath = "C:\Alkane\Alkane.txt"
if ([System.IO.File]::Exists($filePath)) {
Write-Host "File exists at $filePath"
} else {
Write-Host "File does not exist at $filePath"
}
In this script, we use the
[System.IO.File]::Exists()
method to check if the file exists, similar to the
Test-Path
approach. It also prints the appropriate message based on the result.
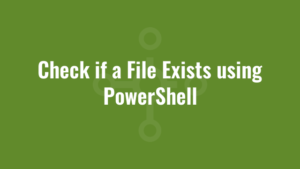
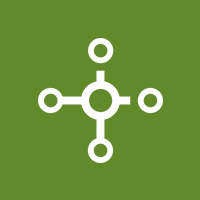