When it comes to making decisions and branching logic in our PowerShell scripts, we commonly use the PowerShell If statement and PowerShell Switch statement.
The PowerShell If Statement
The If
statement is a fundamental control structure in almost every programming language, and PowerShell is no exception. It allows us to execute a block of code based on a condition. Here’s the basic syntax of an If
statement in PowerShell:
if (condition) {
# Code to execute if the condition is true
}
Here’s an example of using an If
statement to check if a number is greater than 10:
$number = 15
if ($number -gt 10) {
Write-Host "The number is greater than 10."
}
In this example, the code within the curly braces will be executed because the condition $number -gt 10
is true.
Common Logical Operators
When working with If
statements, we can use common logical operators to create conditions. Here are some of the most commonly used logical operators:
- Equality (
-eq
): Checks if two values are equal. - Inequality (
-ne
): Checks if two values are not equal. - Greater Than (
-gt
) and Less Than (-lt
): Checks if one value is greater than or less than another value. - Greater Than or Equal To (
-ge
) and Less Than or Equal To (-le
): Checks if one value is greater than or equal to or less than or equal to another value. - Logical AND (
-and
): Checks if multiple conditions are true. - Logical OR (
-or
): Checks if at least one of multiple conditions is true. - Logical NOT (
-not
) or Negation (!
): Reverses the logical value of a condition.
We can combine these logical operators to create complex conditions for our If
statements, allowing us to make decisions based on multiple factors and criteria in our PowerShell scripts. For example:
$number = 15
if ($number -gt 10 -and $number -lt 20) {
Write-Host "The number is greater than 10."
}
The PowerShell Switch Statement
The Switch
statement is a powerful tool for handling multiple conditions in a concise manner. It’s especially useful when we need to perform different actions based on the value of a variable. The Switch
statement can be more readable than a series of nested If
statements.
Here’s the basic syntax of a Switch
statement in PowerShell:
switch (expression) {
value1 { # Code to execute if expression matches value1 }
value2 { # Code to execute if expression matches value2 }
default { # Code to execute if no match is found }
}
Here’s an example of using a Switch
statement to categorize fruit based on its colour:
$fruit = "apple"
switch ($fruit) {
"banana" {
Write-Host "This is a yellow fruit."
}
"apple" {
Write-Host "This is a red fruit."
}
default {
Write-Host "Unknown fruit."
}
}
In this example, the code within the block corresponding to the value “apple” is executed because $fruit
matches “apple.”
The If statement and Switch statement are essential for making decisions in our scripts and can be used in a wide range of scenarios.
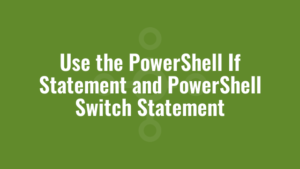
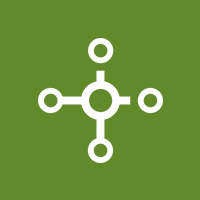