This blog provides a simple example of using PowerShell to read and write XML nodes and attribute names.
Using PowerShell to Write XML
In this example, we first create a new
[System.Xml.XmlDocument]
object to represent our XML document. We then create a root node and append it to the document. We create a child node, add an attribute and inner text, and append it to the root node.
Finally, we save the XML document to a file using the
Save
method.
# Create new XML document
$xml = New-Object -TypeName System.Xml.XmlDocument
# Create root node
$root = $xml.CreateElement("RootNode")
$xml.AppendChild($root)
# Create child node with attribute and value
$child = $xml.CreateElement("ChildNode")
$child.SetAttribute("AttributeName", "AttributeValue")
$child.InnerText = "Inner text"
$root.AppendChild($child)
# Save XML to file
$xml.Save("C:\alkane\example.xml")
Using PowerShell to Read XML
In this example, we first load an XML file using the
Get-Content
cmdlet and cast it as an
[xml]
object using the PowerShell type accelerator. We can then access specific elements and attributes within the XML document using dot notation.
# Load XML file
[xml]$xml = Get-Content -Path C:\alkane\example.xml
# Access XML elements and attributes
$xml.RootNode.ChildNode.AttributeName
$xml.RootNode.ChildNode.InnerText
Using PowerShell to Iterate Through XML Nodes and Attributes
Let’s suppose our XML file has more than one child node like so:
# Create new XML document
$xml = New-Object -TypeName System.Xml.XmlDocument
# Create root node
$root = $xml.CreateElement("RootNode")
$xml.AppendChild($root)
# Create child node with attribute and value
$child = $xml.CreateElement("ChildNode")
$child.SetAttribute("AttributeName", "AttributeValue1")
$child.InnerText = "Inner text 1"
$root.AppendChild($child)
# Create another child node with attribute and value
$child = $xml.CreateElement("ChildNode")
$child.SetAttribute("AttributeName", "AttributeValue2")
$child.InnerText = "Inner text 2"
$root.AppendChild($child)
# Save XML to file
$xml.Save("C:\alkane\example.xml")
We might then want to loop through these child nodes to read each node text and attribute. We can do this like so:
# Load XML file
[xml]$xml = Get-Content -Path C:\alkane\example.xml
# Loop through nodes and attributes
foreach ($node in $xml.RootNode.ChildNodes) {
Write-Host "Node name: $($node.Name)"
Write-Host "Node value: $($node.InnerText)"
foreach ($attribute in $node.Attributes) {
Write-Host "Attribute name: $($attribute.Name)"
Write-Host "Attribute value: $($attribute.Value)"
}
}
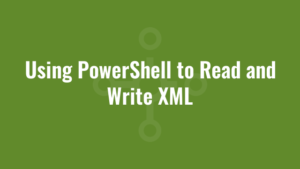
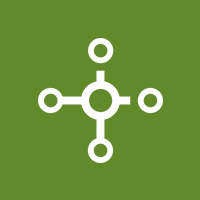