This post contains an example of how we can use PowerShell to display a basic toast notification. If you would like more advanced features, see use PowerShell to display an advanced toast notification.
When we construct a toast notification, we define the way it looks by using XML. And the schema/format of this XML is pre-defined in 8 XML templates:
ToastImageAndText01 | Image, regular text wrapped across 3 lines |
ToastImageAndText02 | Image, bold text on line 1, regular text wrapped across lines 2 and 3 |
ToastImageAndText03 | Image, bold text wrapped across lines 1 and 2, regular text on line 3 |
ToastImageAndText04 | Image, bold text on line 1, regular text on line 2, regular text on line 3 |
ToastText01 | Regular text wrapped across 3 lines |
ToastText02 | Bold text on line 1, regular text wrapped across lines 2 and 3 |
ToastText03 | Bold text wrapped across lines 1 and 2, regular text on line 3 |
ToastText04 | Bold text on line 1, regular text on line 2, regular text on line 3 |
Let’s take ToastTest02 as our simple example. To find out what the default XML schema is for that type of notification we can use the following:
$ToastText02 = [Windows.UI.Notifications.ToastTemplateType, Windows.UI.Notifications, ContentType = WindowsRuntime]::ToastText02
$ToastText02XML = [Windows.UI.Notifications.ToastNotificationManager, Windows.UI.Notifications, ContentType = WindowsRuntime]::GetTemplateContent($ToastText02)
#view the default XML
$ToastText02XML.GetXml()
which will output the following default template:
<toast>
<visual>
<binding template="ToastText02">
<text id="1"></text>
<text id="2"></text>
</binding>
</visual>
</toast>
Now all we need to do is substitute in values for the
<text>
elements. We can easily do this using XPath because the template handily gives each
<text>
element an id attribute of 1 and 2.
$ToastText02 = [Windows.UI.Notifications.ToastTemplateType, Windows.UI.Notifications, ContentType = WindowsRuntime]::ToastText02
$ToastText02XML = [Windows.UI.Notifications.ToastNotificationManager, Windows.UI.Notifications, ContentType = WindowsRuntime]::GetTemplateContent($ToastText02)
$text1 = 'This is some text.'
$text2 = 'And this is more text.'
#replace text with attribute id of 1
$ToastText02XML.SelectSingleNode('//text[@id="1"]').InnerText = $text1
#replace text with attribute id of 2
$ToastText02XML.SelectSingleNode('//text[@id="2"]').InnerText = $text2
#view the updated XML
$ToastText02XML.GetXml()
and the XML now becomes:
<toast>
<visual>
<binding template="ToastText02">
<text id="1">This is some text.</text>
<text id="2">And this is more text.</text>
</binding>
</visual>
</toast>
Each toast notification then needs a specific AppId, and this is important because the name of this application will show in our toast notification. This can be any AppId retrieved from running
Get-StartApps
in PowerShell. As an example, here we use Windows PowerShell as our AppId:
$AppId = (Get-StartApps | Where Name -eq "Windows PowerShell" | Select -First 1 -ExpandProperty AppID)
and here we user Software Center as our AppId:
$AppId = (Get-StartApps | Where Name -eq "Software Center" | Select -First 1 -ExpandProperty AppID)
When we join it all together we can then display a simple toast notification like so:
#get template
$ToastText02 = [Windows.UI.Notifications.ToastTemplateType, Windows.UI.Notifications, ContentType = WindowsRuntime]::ToastText02
$ToastText02XML = [Windows.UI.Notifications.ToastNotificationManager, Windows.UI.Notifications, ContentType = WindowsRuntime]::GetTemplateContent($ToastText02)
#define our text
$text1 = 'This is some text.'
$text2 = 'And this is more text.'
#replace template with out text
$ToastText02XML.SelectSingleNode('//text[@id="1"]').InnerText = $text1
$ToastText02XML.SelectSingleNode('//text[@id="2"]').InnerText = $text2
#retrieve the appid
$AppId = (Get-StartApps | Where Name -eq "Windows PowerShell" | Select -First 1 -ExpandProperty AppID)
#show notification
[Windows.UI.Notifications.ToastNotificationManager]::CreateToastNotifier($AppId).Show($ToastText02XML)
It’s also worth noting that you can only invoke toast notifications as the logged in user – NOT from the system account or otherwise, in which case you will likely get an access denied error.
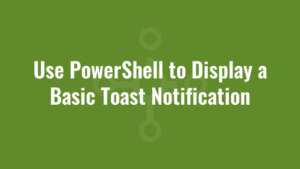
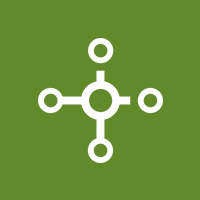