There are various situations where we may need to introduce delays or pauses in our scripts to ensure proper execution or to control the timing of specific actions. Here we demonstrate how to use PowerShell start-sleep to pause script execution.
What is Start-Sleep in PowerShell?
The
Start-Sleep
cmdlet in PowerShell is used to pause the execution of a script or a part of a script for a specified amount of time. This is particularly useful when we need to wait for specific events, give time for a process to complete, or control the timing of script actions.
The basic syntax of
Start-Sleep
is as follows:
Start-Sleep -Seconds <seconds>
Where
<seconds>
is the number of seconds to pause script execution. We can also use the
-Milliseconds
parameter to specify the pause time in milliseconds if more precise timing is required.
Using Start-Sleep in PowerShell
Let’s explore some practical examples of using
Start-Sleep
in PowerShell scripts:
Example 1: Pausing Script Execution
In this example, we’ll use
Start-Sleep
to pause the script for 5 seconds:
# Display a message
Write-Host "Starting a task..."
# Pause script execution for 5 seconds
Start-Sleep -Seconds 5
# Continue the task
Write-Host "Task completed!"
When we run this script, it will display “Starting a task…”, pause for 5 seconds, and then display “Task completed!”.
Example 2: Controlling Loop Timing
Start-Sleep
is particularly useful for controlling the timing of loops. In this example, we’ll use
Start-Sleep
within a loop to introduce a delay between iterations:
# Loop through a collection
$collection = 1..5
foreach ($item in $collection) {
Write-Host "Processing item $item"
# Pause for 2 seconds between iterations
Start-Sleep -Seconds 2
}
With this script, each iteration of the loop will process an item and then pause for 2 seconds before moving on to the next item.
Example 3: Waiting for a Process to Complete
Another common use case for
Start-Sleep
is waiting for something to install before continuing with the script. For example, we might want to wait for the presence of file called C:\Alkane\alkane.txt before we continue our script:
$filePath = "C:\Alkane\alkane.txt"
$loopCount = 5
$loopInterval = 10 # Sleep for 10 seconds in each iteration
for ($i = 1; $i -le $loopCount; $i++) {
Write-Host "Iteration $i - Checking for the existence of the file..."
if (Test-Path $filePath) {
Write-Host "File found! Exiting the loop."
break # Exit the loop when the file is found
}
# Sleep for the specified interval
Start-Sleep -Seconds $loopInterval
}
if ($i -gt $loopCount) {
Write-Host "File not found after $loopCount iterations."
}
The
Start-Sleep
cmdlet in PowerShell is a valuable tool for introducing delays or pauses in our scripts.
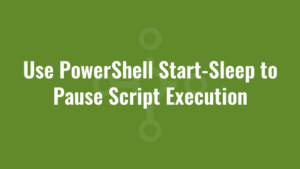
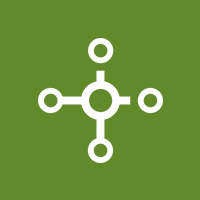