We can use Where-Object in PowerShell scripts to filter objects based on specific conditions.
Where-Object
is commonly used with collections like arrays and lists to extract only the elements that meet certain criteria.
The basic syntax of
Where-Object
is as follows:
collection | Where-Object { condition }
Where:
-
collection
is the array or collection of objects we want to filter. -
condition
is the script block that defines the filtering condition.
Use Where-Object in PowerShell Scripts
Let’s dive into some practical examples to see how
Where-Object
can be used.
Example 1: Filtering Numbers
Suppose we have an array of numbers, and we want to filter out all numbers greater than 10:
$numbers = 1, 5, 12, 8, 15, 3
$filteredNumbers = $numbers | Where-Object { $_ -gt 10 }
# Output the filtered numbers
$filteredNumbers
In this example, the
$_
represents the current item in the collection. We filter the numbers and store the result in
$filteredNumbers
. When we run this script, it will display only the numbers greater than 10.
Example 2: Filtering Files
We can also use
Where-Object
to filter files based on specific criteria. For instance, let’s filter and display all the
.txt
files in a directory:
$files = Get-ChildItem -Path "C:\AlkaneDirectory"
$txtFiles = $files | Where-Object { $_.Extension -eq ".txt" }
# Output the filtered .txt files $txtFiles
In this example,
Get-ChildItem
is used to get a list of files in a directory, and
Where-Object
filters the files based on their extension.
Complex Conditions
Where-Object
is incredibly flexible, allowing us to create complex conditions by combining logical operators, such as
-and
,
-or
, and
-not
. For instance, we can filter a collection based on multiple conditions:
$employees = @(
@{ Name = "John"; Department = "HR"; Salary = 50000 },
@{ Name = "Jane"; Department = "IT"; Salary = 60000 },
@{ Name = "Bob"; Department = "Finance"; Salary = 55000 }
)
# Filter employees in the IT department with a salary greater than £55000
$filteredEmployees = $employees | Where-Object { $_.Department -eq "IT" -and $_.Salary -gt 55000 }
# Output the filtered employees
$filteredEmployees
In this example, we filter employees in the IT department with a salary greater than £55,000 using
-and
to combine the conditions.
Where-Object
offers flexibility in defining conditions, allowing us to work with data in a more efficient and targeted way.
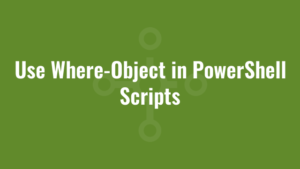
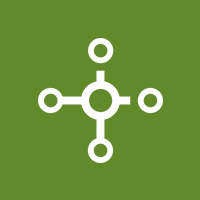