The “Do While” and “Do Until” loops provide valuable options for executing code based on specified conditions. In this blog post, we’ll dive into using the PowerShell Do While loop and Do Until loop, their syntax, and how we can effectively use them in our scripts.
Understanding the Do-While Loop
The “Do While” loop in PowerShell allows us to repeatedly execute a block of code as long as a specified condition remains true. Unlike the While loop, the “Do While” loop always executes the code block at least once before checking the condition for further iterations.
Syntax of the Do-While Loop
The syntax of the “Do While” loop is as follows:
do {
# Code to execute
} while (Condition)
In this structure, the code block within the “Do” block is executed, and then the condition is evaluated. If the condition is true, the loop continues. If the condition is false, the loop exits.
Understanding the PowerShell Do-Until Loop
The “Do Until” loop in PowerShell is similar to the “Do While” loop, with the key difference being that it continues execution until a specified condition becomes true.
Syntax of the Do-Until Loop
The syntax of the “Do Until” loop is as follows:
do {
#Code to execute
} until (Condition)
In this structure, the code block within the “Do” block is executed, and then the condition is evaluated. If the condition is false, the loop continues. If the condition becomes true, the loop exits.
PowerShell Do While Loop and Do Until Loop Examples
Let’s explore examples to illustrate the usage of the “Do While” and “Do Until” loops in PowerShell:
Example 1: Do-While Loop
$count = 1
do {
write-host "Count is: $count"
$count++
} while ($count -le 5)
In this example, we use a “Do While” loop to count from 1 to 5. The loop executes the code block at least once before evaluating the condition for further iterations.
Example 2: Do-Until Loop
$sum = 0
do {
$userInput = Read-Host "Enter a number (or 0 to exit)"
if ($userInput -eq 0) {
break
}
$sum += [int]$userInput
} until ($userInput -eq 0)
write-host "Sum of numbers: $sum"
In this example, we use a “Do Until” loop to continually prompt the user for numbers until they enter 0. The loop executes until the condition (of entering 0) becomes true.
By understanding their syntax and applications, we can effectively use them in our scripts to automate processes and manipulate data with precision.
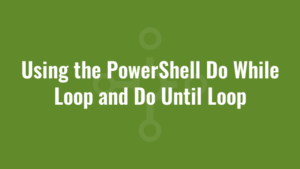
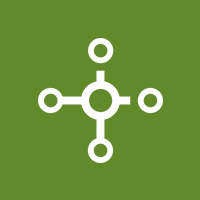