Other Posts in this Series:
- Use ADSI to List Nested Members of an AD Group (Updated)
- Use ADSI and FromFileTime to Convert Datetime Attributes in Active Directory
- Use ADSI to Find Logon Workstations in Active Directory
- Search Active Directory using PowerShell ADSISearcher Filters
- Use PowerShell ADSI to Search Users in Active Directory
This post provides an example of how we can use PowerShell and ADSI to add, update, clear and append Active Directory attributes.
Use Put() to Set an Active Directory Attribute using PowerShell
In its simplest form, we can use the Put()
method to simply set an Active Directory attribute (in this case on a user record) using PowerShell like so:
$objSearcher=[adsisearcher]'(&(objectCategory=person)(objectClass=user)(sAMAccountName=alkaneuser))'
$colProplist = "department"
foreach ($i in $colPropList) { $objSearcher.PropertiesToLoad.Add($i) | out-null }
$colResults = $objSearcher.FindOne()
if ($colResults -ne $null)
{
$user = [adsi]($colResults.Properties).adspath[0]
#set attribute
$user.Put("department", "Example department2");
$user.setinfo();
}
Use PutEx() to Update and Append to an Active Directory Attribute using PowerShell
We can also use the Put()
method to update an Active Directory attribute in similar fashion. But using PutEx()
gives us more flexibility, since we can use it to update, append (for multi-string attribute types), delete and completely clear Active Directory attributes.
$ADS_PROPERTY_CLEAR = 1
$ADS_PROPERTY_UPDATE = 2
$ADS_PROPERTY_APPEND = 3
$ADS_PROPERTY_DELETE = 4
$objSearcher=[adsisearcher]'(&(objectCategory=person)(objectClass=user)(sAMAccountName=alkaneuser))'
$colProplist = "department"
foreach ($i in $colPropList) { $objSearcher.PropertiesToLoad.Add($i) | out-null }
$colResults = $objSearcher.FindOne()
if ($colResults -ne $null)
{
$user = [adsi]($colResults.Properties).adspath[0]
#set attribute
$user.Put("department", "Example1");
$user.setinfo();
#update attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_UPDATE, "department", @("Example2"));
$user.setinfo();
#update attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_DELETE, "department", @("Example2"));
$user.setinfo();
#append attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_APPEND, "department", @("Example3"));
$user.setinfo();
}
Note in the above example that we are updating the department
attribute, which is a single string attribute. Hence if we try and append to it when it is already set, we will see an error (we can’t append to singular strings, only multi-string arrays). So in the example above we delete the attribute first to prevent this from happening. Otherwise we can simply update the attribute instead.
Contrast this with the otherhomephone
attribute, which is a multi-string attribute (essentially an array of strings) that we can append to:
$ADS_PROPERTY_CLEAR = 1
$ADS_PROPERTY_UPDATE = 2
$ADS_PROPERTY_APPEND = 3
$ADS_PROPERTY_DELETE = 4
$objSearcher=[adsisearcher]'(&(objectCategory=person)(objectClass=user)(sAMAccountName=alkaneuser))'
$colProplist = "otherhomephone"
foreach ($i in $colPropList) { $objSearcher.PropertiesToLoad.Add($i) | out-null }
$colResults = $objSearcher.FindOne()
if ($colResults -ne $null)
{
$user = [adsi]($colResults.Properties).adspath[0]
#set attribute
$user.Put("otherhomephone", "Example1");
$user.setinfo();
#update attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_UPDATE, "otherhomephone", @("Example2"));
$user.setinfo();
#append attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_APPEND, "otherhomephone", @("Example3"));
$user.setinfo();
}
Use PutEx() to Clear an Active Directory Attribute using PowerShell
Finally we can clear an Active Directory attribute like so:
$ADS_PROPERTY_CLEAR = 1
$ADS_PROPERTY_UPDATE = 2
$ADS_PROPERTY_APPEND = 3
$ADS_PROPERTY_DELETE = 4
$objSearcher=[adsisearcher]'(&(objectCategory=person)(objectClass=user)(sAMAccountName=alkaneuser))'
$colProplist = "department"
foreach ($i in $colPropList) { $objSearcher.PropertiesToLoad.Add($i) | out-null }
$colResults = $objSearcher.FindOne()
if ($colResults -ne $null)
{
$user = [adsi]($colResults.Properties).adspath[0]
#clear attribute - uncomment as required
$user.PutEx($ADS_PROPERTY_CLEAR, "department", $null);
$user.setinfo();
}
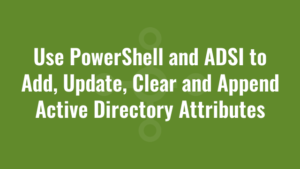
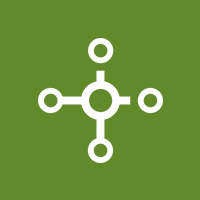